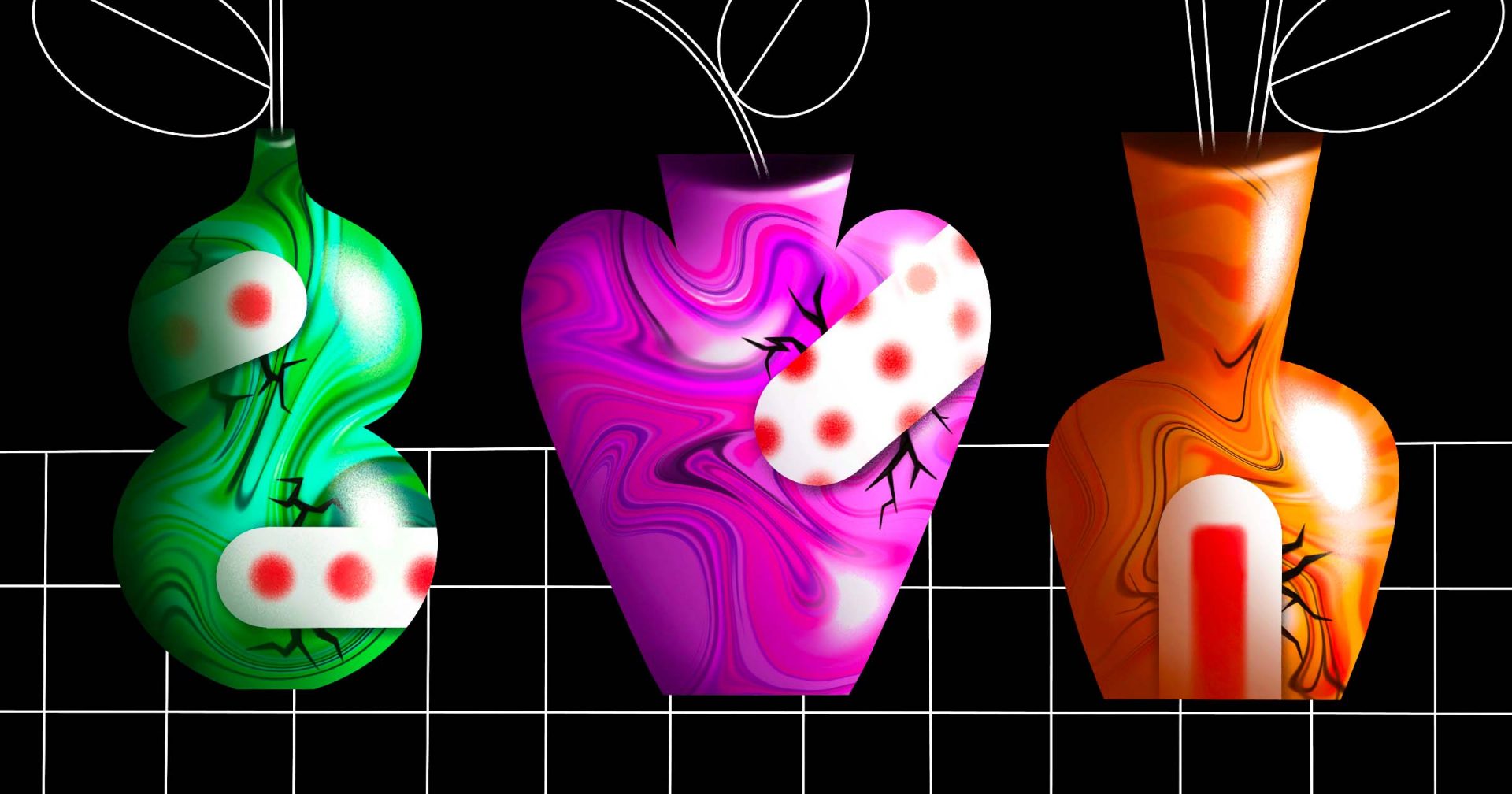
Possess patterns allow you assert reward alternate recommendations to your code
Essentially the most satisfying considerations in tool engineering are of us that no person has solved earlier than. Cracking a special train is something that it’s seemingly you’ll additionally employ in job interviews and notify about in conferences. However the actual fact is that the bulk of challenges you face can salvage already been solved. That you simply can employ these alternate recommendations to higher your have tool.
Instrument carry out patterns are conventional alternate recommendations for the reoccurring carry out considerations in tool engineering. They’re cherish the completely practices employed by many skilled tool builders. That you simply can employ carry out patterns to invent your software program scalable and flexible.
In this text, you’ll behold what carry out patterns are and the map it’s seemingly you’ll additionally assert them to accept better tool capabilities, either from the launch or through refactoring your reward code.
Negate: Earlier than finding out carry out patterns, you wish to salvage a current concept of object-oriented programming.
What are carry out patterns?
Possess patterns are alternate recommendations to steadily taking place carry out considerations in increasing flexible tool the usage of object-oriented programming. Possess patterns on the total employ lessons and objects, but it’s seemingly you’ll additionally also put in pressure just a few of them the usage of functional programming. They outline how lessons wants to be structured and how they should always be in contact with one one more in verbalize to solve explicit considerations.
Some rookies might maybe combine up carry out patterns and algorithms. While an algorithm is a effectively-defined enviornment of instructions, a carry out sample is the next-level description of a solution. That you simply can put in pressure a carry out sample in diverse ways, whereas you salvage to assert the explicit instructions in an algorithm. They don’t solve the train; they solve the carry out of the solution.
Possess patterns are no longer blocks of code it’s seemingly you’ll additionally reproduction and paste to put in pressure. They are cherish frameworks of alternate recommendations with which one can solve a explicit train.
Classification of carry out patterns
The book, Possess Patterns- Parts of Reusable Object-Oriented Instrument written by the Gang of Four (Erich Gamma, John Vlissides, Ralph Johnson, and Richard Helm) offered the premise of carry out patterns in tool sort. The book contains 23 carry out patterns to solve a fluctuate of object-oriented carry out considerations. These patterns are a toolbox of tried and examined alternate recommendations for diverse traditional considerations that it’s seemingly you’ll come across whereas increasing tool capabilities.
Possess patterns fluctuate in step with their complexity, level of detail, and scope of applicability for the total system. They’re going to even be labeled into three groups in step with their motive:
- Creational patterns recount diverse solutions for creating objects to amplify code flexibility and reuse.
- Structural patterns recount family between objects and lessons in making them into complicated buildings whereas keeping them flexible and efficient.
- Behavioral patterns outline how objects should always be in contact and engage with one one more.
Why whenever you occur to make employ of carry out patterns?
You on the total is a official tool developer even for of us that don’t know a single carry out sample. It’s seemingly you’ll be the usage of some carry out patterns with out even vivid them. However vivid carry out patterns and how one can employ them will give you an opinion of solving a particular train the usage of the completely carry out tips of object-oriented programming. That you simply can refactor complicated objects into more effective code segments that are easy to put in pressure, regulate, test, and reuse. You don’t should always confine yourself to 1 explicit programming language; it’s seemingly you’ll additionally put in pressure carry out patterns in any programming language. They record the premise, no longer the implementation.
Possess patterns are all referring to the code. They invent you assert the completely carry out tips of tool sort, such because the originate/closed opinion (objects wants to be originate for extension but closed for modification) and the single responsibility opinion (A class must salvage completely one cause to commerce). This article discusses carry out tips in higher detail.
That you simply can invent your software program more flexible by the usage of carry out patterns that break it into reusable code segments. That you simply can add new functions to your software program with out breaking the reward code at any time. Possess patterns also enhance the readability of code; if anyone wants to increase your software program, they’re going to grab the code with runt train.
What are worthwhile carry out patterns?
Each and each carry out sample solves a explicit train. That you simply can employ it in that person train. When you make employ of carry out patterns in the atrocious context, your code looks complicated, with many lessons and objects. The following are some examples of potentially the most steadily dilapidated carry out patterns.
Singleton carry out sample
Object oriented code has a wicked reputation for being cluttered. How are you going to steer certain of making great numbers of pointless objects? How are you going to limit the different of conditions of a class? And the map can a class relief watch over its instantiation?
Using a singleton sample solves these considerations. It’s a creational carry out sample that describes how one can outline lessons with completely a single occasion which can be accessed globally. To place in pressure the singleton sample, it’s top to invent the constructor of the major class non-public so as that it’s completely accessible to participants of the class and invent a static map (getInstance
) for object advent that acts as a constructor.
Right here’s the implementation of the singleton sample in Python.
# Implementation of the Singleton Pattern
class Singleton(object):
_instance = None
def __init__(self):
elevate RuntimeError('Call getInstance() as a replace')
@classmethod
def getInstance(cls):
if cls._instance is None:
print('Creating the object')
cls._instance = great().__new__(cls)
return cls._instance
The above code is the aged technique to put in pressure the singleton sample, but it’s seemingly you’ll additionally invent it more straightforward by the usage of __new__
or making a metaclass
).
It’s top to employ this carry out sample completely for of us that are 100% certain that your software program requires completely a single occasion of the major class. Singleton sample has loads of drawbacks in contrast to diverse carry out patterns:
- You mustn’t outline something in the world scope but singleton sample affords globally accessible occasion.
- It violates the Single-responsibility opinion.
Study out some more drawbacks of the usage of a singleton sample.
Decorator carry out sample
Should always you’re following SOLID tips (and in traditional, it’s top to), you’ll should always invent objects or entities that are originate for extension but closed for modification. How are you going to lengthen the performance of an object at bustle-time? How are you going to lengthen an object’s behavior with out affecting the more than a few reward objects?
That you simply can relief in tips the usage of inheritance to increase the behavior of an reward object. Then all all over again, inheritance is static. That you simply can’t regulate an object at runtime. Alternatively, it’s seemingly you’ll additionally employ the decorator sample to add extra performance to objects (subclasses) at runtime with out altering the parent class. The decorator sample (on occasion called a wrapper) is a structural carry out sample that permits you to duvet an reward class with loads of wrappers.
For wrappers, it employs summary lessons or interfaces through composition (in resolution to inheritance). In composition, one object contains an occasion of diverse lessons that put in pressure the desired performance rather than inheriting from the parent class. Many carry out patterns, including the decorator, are in step with the opinion of composition. Study out why it’s top to employ composition over inheritance.
# Imposing decorator sample
class Element():
def operation(self):
proceed
class ConcreteComponent(Element):
def operation(self):
return 'ConcreteComponent'
class Decorator(Element):
_component: Element = None
def __init__(self, part: Element):
self._component = part
@property
def part(self):
return self._component
def operation(self):
return self._component.operation()
class ConcreteDecoratorA(Decorator):
def operation(self):
return f"ConcreteDecoratorA({self.part.operation()})"
class ConcreteDecoratorB(Decorator):
def operation(self):
return f"ConcreteDecoratorB({self.part.operation()})"
simpleComponent = ConcreteComponent()
print(simpleComponent.operation())
# decorators can wrap easy components moreover to the more than a few decorators also.
decorator1 = ConcreteDecoratorA(easy)
print(decorator1.operation())
decorator2 = ConcreteDecoratorB(decorator1)
print(decorator2.operation())
The above code is the classic map of enforcing the decorator sample. That you simply can additionally put in pressure it the usage of capabilities.
The decorator sample implements the single-responsibility opinion. That you simply can split great lessons into loads of little lessons, each enforcing a explicit behavior and lengthen them afterward. Wrapping the decorators with diverse decorators increases the complexity of code with loads of layers. Furthermore, it’s no longer easy to salvage away a explicit wrapper from the wrappers’ stack.
Approach carry out sample
How are you going to commerce the algorithm on the bustle-time? That you simply can are seemingly to employ conditional statements. However for of us that’ve many variants of algorithms, the usage of conditionals makes our major class verbose. How are you going to refactor these algorithms to be less verbose?
The technique sample permits you to commerce algorithms at runtime. That you simply can steer certain of the usage of conditional statements contained in the major class and refactor the code into separate technique lessons. Within the technique sample, it’s top to stipulate a family of algorithms, encapsulate each and invent them interchangeable at runtime.
That you simply can with out train put in pressure the technique sample by creating separate lessons for algorithms. That you simply can additionally put in pressure diverse solutions as capabilities in resolution to the usage of lessons.
Right here’s a conventional implementation of the technique sample:
# Imposing technique sample
from abc import ABC, abstractmethod
class Approach(ABC):
@abstractmethod
def enact(self):
proceed
class ConcreteStrategyA(Approach):
def enact(self):
return "ConcreteStrategy A"
class ConcreteStrategyB(Approach):
def enact(self):
return "ConcreteStrategy B"
class Default(Approach):
def enact(self):
return "Default"
class Context:
technique: Approach
def setStrategy(self, technique: Approach = None):
if technique is no longer None:
self.technique = technique
else:
self.technique = Default()
def executeStrategy(self):
print(self.technique.enact())
## Instance software program
appA = Context()
appB = Context()
appC = Context()
appA.setStrategy(ConcreteStrategyA())
appB.setStrategy(ConcreteStrategyB())
appC.setStrategy()
appA.executeStrategy()
appB.executeStrategy()
appC.executeStrategy()
Within the above code snippet, the customer code is easy and simple. However in exact-world software program, the context changes rely upon particular person actions, cherish after they click on a button or commerce the extent of the recreation. For instance, in a chess software program, the computer uses diverse technique for of us that resolve the extent of train.
It follows the single-responsibility opinion because the huge explain material major (context
) class is split into diverse technique lessons. That you simply can add as many extra solutions as you like whereas keeping the major class unchanged (originate/closed opinion). It increases the flexibility of our software program. It will be completely to employ this sample when your major class has many conditional statements that swap between diverse variants of the same algorithm. Then all all over again, in case your code contains completely just a few algorithms, there is no should always employ a technique sample. It lawful makes your code peruse complicated with all the teachings and objects.
Enlighten carry out sample
Object oriented programming in particular has to take care of the exclaim that the software program is currently in. How are you going to commerce an object’s behavior in step with its inside exclaim? What’s the completely technique to stipulate exclaim-explicit behavior?
The exclaim sample is a behavioral carry out sample. It affords an different formulation to the usage of large conditional blocks for enforcing exclaim-dependent behavior on your major class. Your software program behaves in a completely different map looking out on its inside exclaim, which a particular person can commerce at runtime. That you simply can carry out finite exclaim machines the usage of the exclaim sample. Within the exclaim sample, it’s top to stipulate separate lessons for each exclaim and add transitions between them.
# enforcing exclaim sample
from __future__ import annotations
from abc import ABC, abstractmethod
class Context:
_state = None
def __init__(self, exclaim: Enlighten):
self.setState(exclaim)
def setState(self, exclaim: Enlighten):
print(f"Context: Transitioning to {variety(exclaim).__name__}")
self._state = exclaim
self._state.context = self
def doSomething(self):
self._state.doSomething()
class Enlighten(ABC):
@property
def context(self):
return self._context
@context.setter
def context(self, context: Context):
self._context = context
@abstractmethod
def doSomething(self):
proceed
class ConcreteStateA(Enlighten):
def doSomething(self):
print("The context is in the exclaim of ConcreteStateA.")
print("ConcreteStateA now changes the exclaim of the context.")
self.context.setState(ConcreteStateB())
class ConcreteStateB(Enlighten):
def doSomething(self):
print("The context is in the exclaim of ConcreteStateB.")
print("ConcreteStateB wants to commerce the exclaim of the context.")
self.context.setState(ConcreteStateA())
# instance software program
context = Context(ConcreteStateA())
context.doSomething()
context.doSomething()
Enlighten sample follows both the single-responsibility opinion moreover to the originate/closed opinion. That you simply can add as many states and transitions as you like with out altering the major class. The exclaim sample is terribly an associated to the technique sample, but a technique is blind to diverse solutions, whereas a exclaim is responsive to diverse states and can swap between them. If your class (or exclaim machine) has just a few states or infrequently ever changes, it’s top to steer certain of the usage of the exclaim sample.
Negate carry out sample
The record sample is a behavioral carry out sample that encapsulates the total info just a few search files from exact into a separate record
object. Using the record sample, it’s seemingly you’ll additionally store loads of instructions in a class to employ them repeatedly. It permits you to parameterize solutions with diverse requests, delay or queue a search files from’s execution, and make stronger undoable operations. It increases the flexibility of your software program.
A record sample implements the single-responsibility opinion, as you salvage divided the search files from into separate lessons corresponding to invokers
, instructions
, and receivers
. It also follows the originate/closed opinion. That you simply can add new record objects with out altering the earlier instructions.
Command you wish to put in pressure reversible operations (cherish undo/redo) the usage of a record sample. If that is so, it’s top to relief a record history: a stack containing all accomplished record objects and the software program’s exclaim. It consumes a bunch of RAM, and barely it’s very unlikely to put in pressure an efficient solution. It’s top to employ the record sample for of us that’ve many instructions to enact; in another case, the code might maybe change into more complicated since you’re adding a separate layer of instructions between senders and receivers.
Conclusion
Based fully on most tool carry out tips including the effectively-established SOLID tips, it’s top to write down reusable code and extendable capabilities. Possess patterns allow you accept flexible, scalable, and maintainable object-oriented tool the usage of completely practices and carry out tips. The total carry out patterns are tried and examined alternate recommendations for diverse routine considerations. Even for of us that don’t employ them lawful away, vivid about them will give you a closer concept of how one can solve diverse forms of considerations in object-oriented carry out. That you simply can put in pressure the carry out patterns in any programming language as they are lawful the outline of the solution, no longer the implementation.
Should always you’re going to invent great-scale capabilities, it’s top to relief in tips the usage of carry out patterns because they give a closer map of increasing tool. Should always you’re in attending to know these patterns better, relief in tips enforcing each carry out sample on your favourite programming language.
Tags: carry out patterns, tool sort