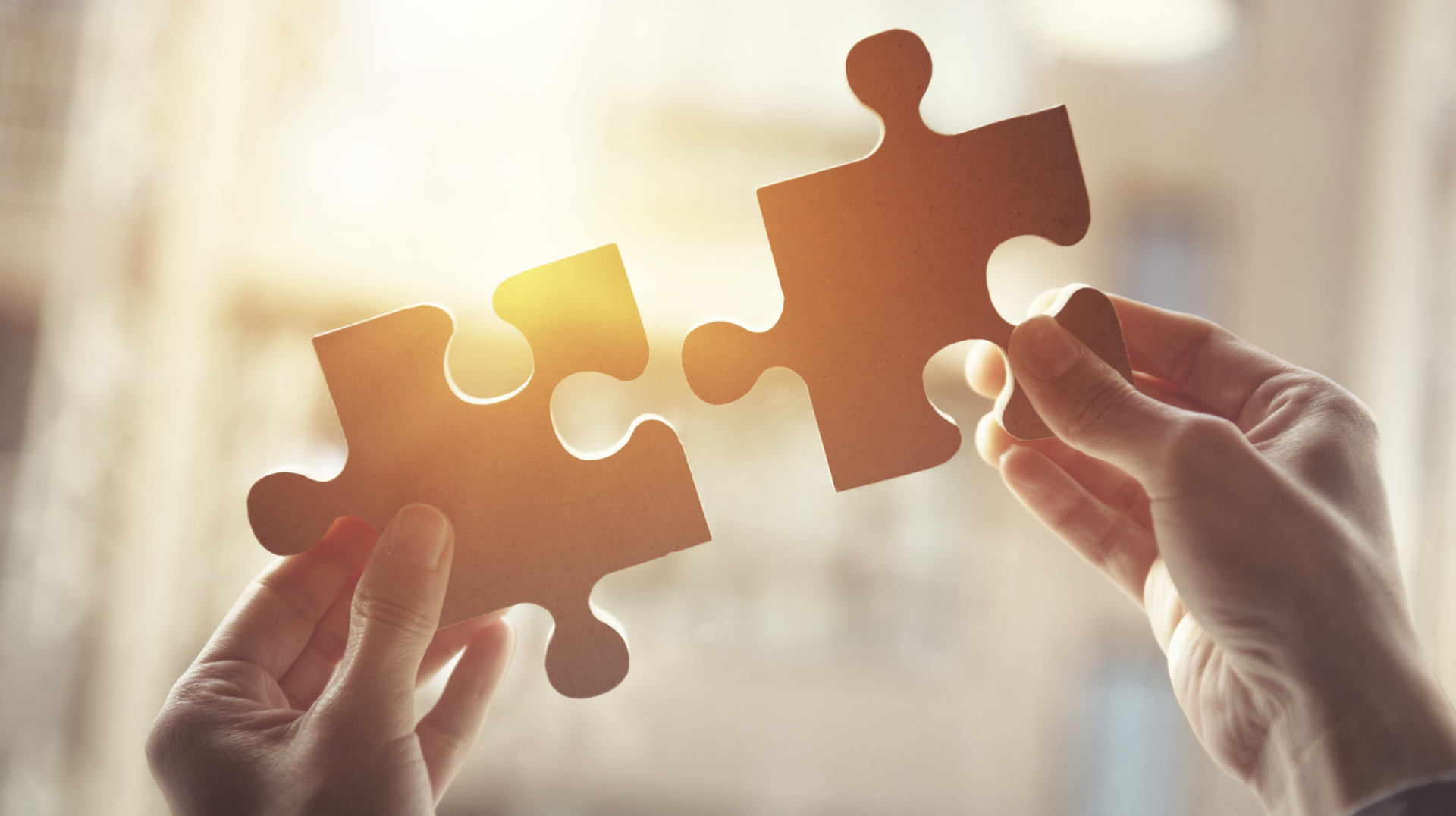
What’s an “Impedance Mismatch” in Programming?
James Walker
| 4 min read

A programming impedance mismatch happens when details desires to be remodeled into a varied architectural paradigm. Essentially the most great example involves object-oriented codebases and relational databases.
An impedance mismatch arises when details is fetched from or inserted into a database. The properties of objects or classes all via the codebase may likely well neutral gentle be mapped to their corresponding database desk fields.
Mapping and Relationships
Your classes received’t necessarily diagram with out lengthen to particular person database tables. The vogue of an object may likely well require details from quite quite a bit of tables to be old kind in aggregate.
You’ll additionally must handle relationships between your details. Relational databases diagram this straight forward by permitting you to reference varied records. It is likely you’ll likely well employ a JOIN
to entry the total details encapsulated by the connection.
CREATE TABLE productTypes( ProductTypeUuid VARCHAR(32) PRIMARY KEY, ProductTypeName VARCHAR(255) NOT NULL UNIQUE ); CREATE TABLE merchandise( ProductUuid VARCHAR(32) PRIMARY KEY, ProductName VARCHAR(255) NOT NULL UNIQUE, ProductType VARCHAR(32) NOT NULL, FOREIGN KEY (ProductType) REFERENCES productTypes(ProductTypeUuid) ON DELETE CASCADE );
The utilization of undeniable SQL, it is likely you’ll likely catch the blended properties of a Product and its ProductType with this straightforward quiz:
SELECT * FROM merchandise INNER JOIN productTypes ON ProductTypeUuid = ProductType;
Then properties of the Product and its ProductType are accessed from the the same flat construction:
echo $file["ProductName"]; echo $file["ProductTypeName"];
This flat array rapidly turns into limiting in complex purposes. Developers naturally model the Product and ProductType entities as separate classes. The Product class may likely well then support an occasion of a ProductType. Here’s how that seems:
closing class ProductType { public characteristic __construct( public string $Uuid, public string $Title) {} } closing class Product { public characteristic __construct( public string $Uuid, public string $Title, public ProductType $ProductType) {} }
There’s now a fundamental impedance mismatch within the code. Some bear of specialized mapping is required sooner than records from the database quiz can even be represented as Product
conditions.
Further issues arise whenever it’s good to entry the total merchandise of a convey form. Here’s the kind it is likely you’ll likely diagram that in code:
closing class ProductType { public characteristic __construct( public string $Uuid, public string $Title, ProductCollection $Merchandise) {} }
Now the ProductType
holds a ProductCollection
, which would maybe likely within the waste accept as true with an array of Product
conditions. This creates a bi-directional relational reference – ProductType
contains all its merchandise and every Product
contains its product form.
This bear of modelling doesn’t exist all via the relational paradigm. Each and every bear of connection is represented with a single relational link file. The employ of bi-directional references simplifies developer entry to object properties. Alternatively, it requires extra complex mapping when transferred to and from the database. Here’s in consequence of SQL doesn’t natively understand the semantics of the model.
Taking into consideration Hierarchy
The model outlined above creates a hierarchy within the codebase: Product
sits below ProductType
. This sounds logical and matches our expectations of the categorical world.
Relational databases don’t recognize hierarchies. As all relationships are the same, relational databases bear an inherently “flat” construction. We saw this earlier when fetching details with a JOIN
.
The shortcoming of hierarchy in SQL methodology all tables have an the same priority to every varied. An cease of right here is that it is likely you’ll likely with out effort entry the properties of records nested deep on your logical object hierarcy. Furthermore, there’s a decrease possibility of cyclical dependencies.
The instance above shows that Product
and ProductType
can cease up relating to every varied in code; the flat nature of relational databases would quit this convey example going on. Cycles can gentle slit up in undeniable SQL however you’re less likely to come serve across them than when modelling with object-oriented code.
Object-oriented programming relies on the composition of straight forward objects into extra complex ones. Relational objects don’t bear the kind of thought of composition or the “straightforward” and “complex” – any file can reference any varied.
Inheritance
Yet every other OOP-queer characteristic is inheritance. It is standard for a class to prolong every other, including extra behaviours. Relational databases are unable to replicate this. It’s not likely for a desk to “prolong” every other desk.
A codebase that uses inheritance will uncover difficulties when child objects are persisted or hydrated by task of a relational database. Throughout the database, you’ll assuredly need two tables. One stores the properties of the depraved object (that you simply’re extending), with every other handling the properties of the baby.
The mapping code then iterates the total properties of the object. Properties deriving from the prolonged class will likely be inserted into the predominant code. Those with out lengthen outlined on the baby will cease up within the 2nd desk.
A the same diagram is required when mapping serve to the codebase from the database. A SQL JOIN
will likely be old kind to catch all records an a lot just like the depraved (prolonged) class, with the baby properties integrated. Those records that contained the baby properties would then be mapped to child class conditions.
CREATE TABLE guardian(Identification INTEGER PRIMARY KEY, A INTEGER); CREATE TABLE child(Identification INTEGER PRIMARY KEY, B INTEGER, ParentId INTEGER);
class Father or mother { public characteristic __construct(int $A) {} } closing class Baby extends Father or mother { public characteristic __construct(int $A, int $B) {} } // Get records // SELECT FROM guardian INNER JOIN child ON child.ParentId = guardian.Identification; $objs = []; foreach ($records as $file) { if (isset($file["B"])) { $objs[] = new Baby($file["A"], $file["B"]); } else $objs[] = new Father or mother($file["A"]); }
The introduction of inheritance necessitates the utilization of extra involving mapping common sense. The incapability of relational databases to model the inheritance capabilities of object-oriented languages introduces this impedance mismatch.
Visibility and Encapsulation
A elementary tenet of object-oriented programming is visibility and encapsulation. Properties are declared as public
or inside of most
. An object’s internal representation can even be hid within the serve of an interface that pre-formats details for the user.
Relational databases lack these controls. There’s no formulation to utter a field as inside of most
, nor is there an glaring must diagram so. Each and every share of details saved in a database has its have honest; there desires to be no redundancy.
This isn’t necessarily staunch when transposed to an object-oriented paradigm. An object may likely well employ two database fields in aggregate to utter a new share of computed knowledge. The values of the 2 particular person fields may likely well be inappropriate to the application and consequently hidden from model.
CREATE TABLE merchandise(Trace INTEGER, TaxRate INTEGER);
closing class Product { public characteristic __construct( stable int $Trace, stable int $TaxRate) {} public characteristic getTotalPrice() : int { return ($this -> Trace * ($this -> TaxRate / 100)); } }
The application handiest cares relating to the total product imprint, including taxes. The unit imprint and tax rate are encapsulated by the Product
class. Visibility controls (stable
) cloak the values. The overall public interface features a single methodology that uses the 2 fields to compute a new imprint.
Extra assuredly, object-oriented programming advocates programming to interfaces. Yelp entry to properties is sorrowful. The utilization of class solutions that put in power an interface permits different implementations to be constructed in some unspecified time in the future.
There may be no say counterpart to this within the relational world. Database views diagram provide a means to mix tables and abstract fields into new forms. You’re gentle working with out lengthen with field values even supposing.
Summary
Object-relational impedance mismatches happen when an object-oriented codebase exchanges details with a relational database. There are elementary variations within the kind whereby details is modelled. This neccessitates the introduction of a mapping layer that transposes details between the formats.
The impedance mismatch train is with out doubt one of many predominant motivating factors within the adoption of ORMs within object-oriented languages. These enable the computerized hydration of complex codebase objects from relational details sources. With out a devoted details mapping layer, handiest basically the most sharp of purposes will bear a straightforward pathway to and from a relational database.