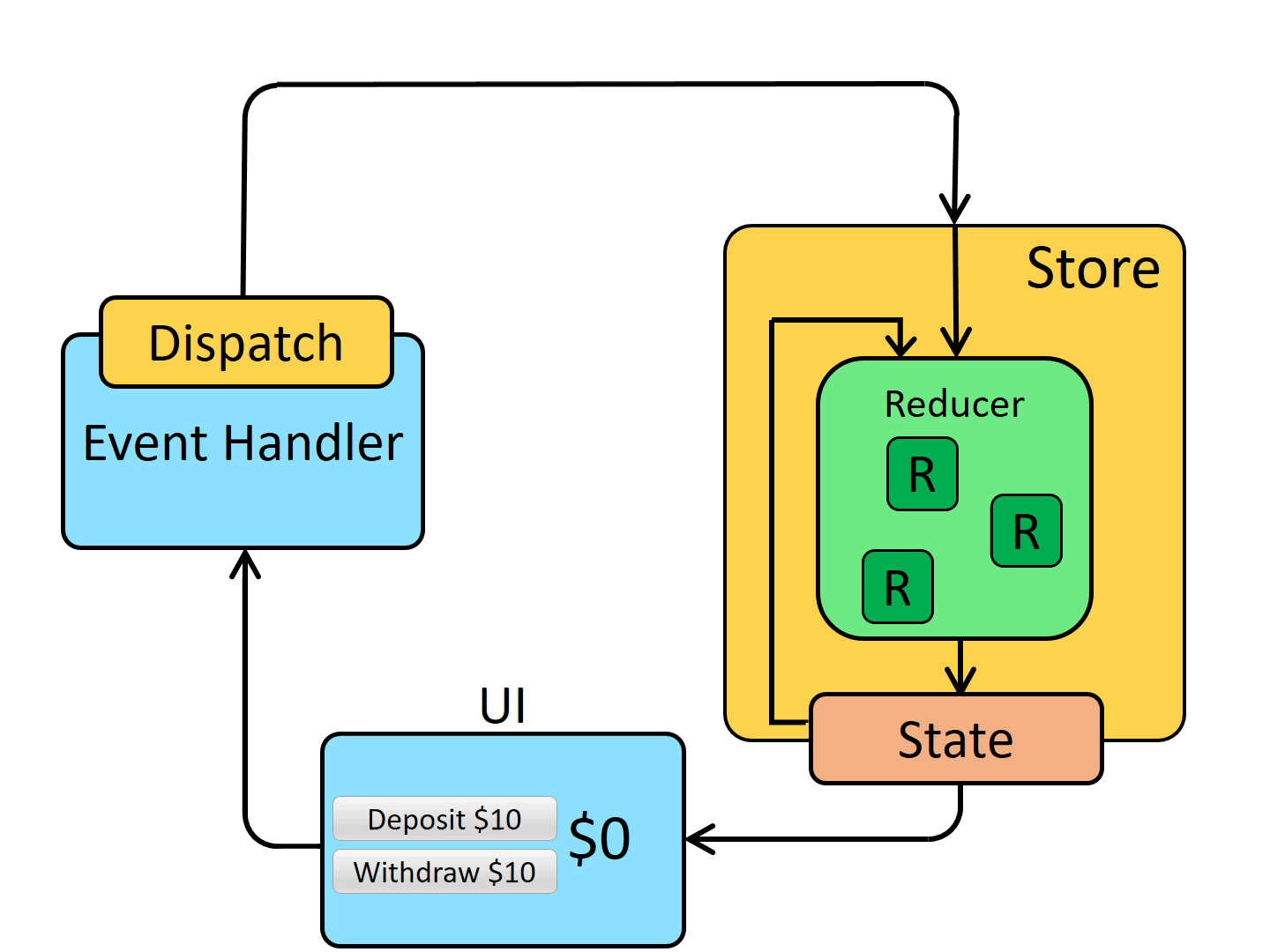
Redux Overview and Ideas – Reimagined Redux Tutorial
What You might maybe presumably Learn
- What Redux is and why it’s doubtless you’ll presumably maybe presumably also opt to make utilize of it
- Key Redux phrases and concepts
- How recordsdata flows thru a Redux app
Introduction
Welcome to the Redux Essentials tutorial! This tutorial will introduce you to Redux and educate you pointers on how to make utilize of it the upright system, the utilize of our most modern in actual fact useful tools and most productive practices. By the point you enact, are attempting so as to originate building your receive Redux applications the utilize of the tools and patterns you come by learned here.
In Part 1 of this tutorial, we will duvet the principle concepts and phrases you come by to know to make utilize of Redux, and in Part 2 we will explore a total React + Redux app to leer how the pieces fit collectively.
Starting in Part 3, we will utilize that recordsdata to construct a puny social media feed app with some steady-world functions, leer how those pieces in truth work in educate, and discuss some essential patterns and pointers for the utilize of Redux.
Learn This Tutorial
This page will point of curiosity on showing you how to make utilize of Redux the upright system, and existing excellent ample of the concepts so that it’s doubtless you’ll presumably maybe presumably also sign pointers on how to construct Redux apps because it’d be.
We come by tried to buy these explanations newbie-pleasant, nonetheless we attain opt to construct some assumptions about what you realize already:
Whenever you happen to’re now now not already pleased with those subjects, we encourage you to buy some time to turn into pleased with them first, and then come encourage to seek out out about Redux. We will be here need to you’re ready!
You should to make sure you come by the React and Redux DevTools extensions establish in to your browser:
- React DevTools Extension:
- Redux DevTools Extension:
What is Redux?
It helps to fancy what this “Redux” thing is in the predominant online page online. What does it attain? What considerations does it encourage me solve? Why would I opt to make utilize of it?
Redux is a pattern and library for managing and updating software assert, the utilize of events called “actions”. It serves as a centralized store for assert that needs to be extinct true thru your complete software, with principles guaranteeing that the assert can easiest be updated in a predictable sort.
Why Would possibly maybe presumably also serene I Exercise Redux?
Redux helps you arrange “world” assert – assert that is mandatory true thru many parts of your software.
The patterns and tools supplied by Redux build it more straightforward to fancy when, where, why, and how the assert to your software is being updated, and how your software common sense will behave when those changes happen. Redux guides you in opposition to writing code that is predictable and testable, which helps give you self perception that your software will work as expected.
When Would possibly maybe presumably also serene I Exercise Redux?
Redux helps you take care of shared assert management, nonetheless fancy quite loads of tool, it has tradeoffs. There might maybe be more concepts to learn, and more code to jot down. It moreover adds some indirection to your code, and asks you to coach certain restrictions. It’s miles a alternate-off between quick term and future productivity.
Redux is more priceless when:
- You come by gigantic quantities of software assert which would perhaps presumably maybe presumably be mandatory in quite loads of locations in the app
- The app assert is updated veritably over time
- The common sense to interchange that assert might maybe presumably just be complex
- The app has a medium or gigantic-sized codebase, and might maybe presumably just be worked on by many folk
Now not all apps need Redux. Clutch some time to evaluate of the form of app you’re building, and decide what tools might maybe presumably maybe be most productive to encourage solve the considerations you’re working on.
Redux Libraries and Instruments
Redux is a puny standalone JS library. Nonetheless, it’s veritably extinct with quite loads of different applications:
React-Redux
Redux can mix with any UI framework, and is most veritably extinct with React. React-Redux is our reputable package deal that lets your React substances work along with a Redux store by reading pieces of assert and dispatching actions to interchange the shop.
Redux Toolkit
Redux Toolkit is our in actual fact useful scheme for writing Redux common sense. It contains applications and capabilities that we judge are mandatory for building a Redux app. Redux Toolkit builds in our urged most productive practices, simplifies most Redux tasks, prevents total mistakes, and makes it more straightforward to jot down Redux applications.
Redux DevTools Extension
The Redux DevTools Extension reveals a historical previous of the changes to the assert to your Redux store over time. This ability that that you just can debug your applications successfully, at the side of the utilize of unprecedented methods fancy “time-trail debugging”.
Redux Terms and Ideas
Sooner than we dive into some steady code, let’s discuss a number of the phrases and concepts it’s doubtless you’ll presumably opt to know to make utilize of Redux.
Deliver Management
Let’s originate by having an indication at a puny React counter part. It tracks a quantity in part assert, and increments the amount when a button is clicked:
characteristic Counter() {
const [counter, setCounter] = useState(0)
const increment = () => {
setCounter(prevCounter => prevCounter + 1)
}
return (
<div>
Price: {counter} <button onClick={increment}>Incrementbutton>
div>
)
}
It’s miles a self-contained app with the following parts:
- The assert, the provision of truth that drives our app;
- The see, a declarative description of the UI in accordance to the most modern assert
- The actions, the events that happen in the app in accordance to person enter, and trigger updates in the assert
Right here’s a puny instance of “one-system recordsdata drift”:
- Deliver describes the location of the app at a particular point in time
- The UI is rendered in accordance to that assert
- When one thing occurs (just like a person clicking a button), the assert is updated in accordance to what took place
- The UI re-renders in accordance to the recent assert
Nonetheless, the simplicity can shatter down as soon as we come by more than one substances that opt to portion and utilize the identical assert, especially if those substances would be found in varied parts of the software. Normally this could be solved by “lifting assert up” to parent substances, nonetheless that would now not constantly encourage.
One system to unravel here’s to extract the shared assert from the substances, and establish it into a centralized online page online outside the part tree. With this, our part tree becomes a immense “see”, and any part can entry the assert or trigger actions, no topic where they are in the tree!
By defining and keeping apart the concepts serious about assert management and implementing principles that buy independence between views and states, we give our code more structure and maintainability.
Right here’s the basic belief on the encourage of Redux: a single centralized online page online to own the area assert to your software, and speak patterns to coach when updating that assert to construct the code predictable.
Immutability
“Mutable” methodology “irritable”. If one thing is “immutable”, it might maybe most likely presumably maybe never be changed.
JavaScript objects and arrays are all mutable by default. If I receive an object, I will alternate the contents of its fields. If I receive an array, I will alternate the contents as smartly:
const obj = { a: 1, b: 2 }
obj.b = 3
const arr = [‘a’, ‘b’]
arr.push(‘c’)
arr[1] = ‘d’
Right here’s called mutating the object or array. It’s the identical object or array reference in memory, nonetheless now the contents inner the object come by changed.
In describe to interchange values immutably, your code need to construct copies of existing objects/arrays, and then modify the copies.
We can attain this by hand the utilize of JavaScript’s array / object spread operators, as smartly as array methods that return recent copies of the array as a replace of mutating the authorized array:
const obj1 = {
a: {
c: 3
}
b: 2,
}
const obj2 = {
…obj
a: {
…obj.a,
c: 42
}
}
const arr = [“a”, “b”];
const arr2 = arr.concat(“c”);
const arr3 = arr.slice();
arr3.push(“c”);
Redux expects that all assert updates are executed immutably. We will sign at where and how here’s essential a chunk later, as smartly as some more straightforward methods to jot down immutable change common sense.
Terminology
There might maybe be some essential Redux phrases that it’s doubtless you’ll presumably opt to be accustomed to before we continue:
Actions
An action is a undeniable JavaScript object that has a variety
discipline. You might maybe presumably maybe presumably judge of an action as an match that describes one thing that took online page online in the software.
The variety
discipline need to serene be a string that provides this action a descriptive name, fancy "todos/todoAdded"
. We most steadily write that variety string fancy "domain/eventName"
, where the predominant segment is the characteristic or class that this action belongs to, and the second segment is the speak thing that took online page online.
An action object can come by other fields with extra information about what took online page online. By convention, we establish that recordsdata in a discipline called payload
.
A conventional action object might maybe presumably sign fancy this:
const addTodoAction = {
variety: ‘todos/todoAdded’,
payload: ‘Rob milk’
}
Circulate Creators
An action creator is a characteristic that creates and returns an action object. We in total utilize these so we develop now now not need to jot down the action object by hand every time:
const addTodo = text => {
return {
variety: ‘todos/todoAdded’,
payload: text
}
}
Reducers
A reducer is a characteristic that receives the most modern assert
and an action
object, decides pointers on how to interchange the assert if the largest, and returns the recent assert: (assert, action) => newState
.
recordsdata
“Reducer” capabilities get their name on fable of they’re just just like the form of callback characteristic you tear to the Array.minimize()
scheme.
Reducers need to constantly educate some speak principles:
- They need to serene easiest calculate the recent assert sign in accordance to the
assert
andaction
arguments - They receive now now not appear to be allowed to change the unusual
assert
. As a replace, they need to construct immutable updates, by copying the unusualassert
and making changes to the copied values. - They need to now now not attain any asynchronous common sense, calculate random values, or cause other “facet effects”
We will talk more about the ideas of reducers later, at the side of why they’re essential and pointers on how to coach them because it’d be.
The common sense inner reducer capabilities in total follows the identical series of steps:
- Check to leer if the reducer cares about this action
- In that case, build a duplicate of the assert, change the copy with recent values, and return it
- In any other case, return the unusual assert unchanged
Right here’s a puny instance of a reducer, showing the steps that every reducer need to serene educate:
const initialState = { sign: 0 }
characteristic counterReducer(assert = initialState, action) {
if (action.variety === ‘counter/increment’) {
return {
…assert,
sign: assert.sign + 1
}
}
return assert
}
Reducers can utilize any form of common sense inner to make a decision what the recent assert need to serene be: if/else
, switch
, loops, and so on.
Detailed Explanation: Why Are They Called ‘Reducers?’
The Array.minimize()
scheme lets you buy an array of values, process every merchandise in the array one after the other, and return a single . You might maybe presumably maybe presumably judge of it as “reducing the array the total system down to one sign”.
Array.minimize()
takes a callback characteristic as an argument, which would perhaps presumably well be called one time for every merchandise in the array. It takes two arguments:
previousResult
, the sign that your callback returned final timecurrentItem
, the most modern merchandise in the array
The first time that the callback runs, there might maybe be never always a previousResult
accessible, so we must always moreover tear in an initial sign that can be extinct as the predominant previousResult
.
If we wanted so as to add collectively an array of numbers to seek out out what the total is, shall we write a minimize callback that looks fancy this:
const numbers = [2, 5, 8]
const addNumbers = (previousResult, currentItem) => {
console.log({ previousResult, currentItem })
return previousResult + currentItem
}
const initialValue = 0
const complete = numbers.minimize(addNumbers, initialValue)
console.log(complete)
Sight that this addNumber
“minimize callback” characteristic would now not opt to buy music of the relaxation itself. It takes the previousResult
and currentItem
arguments, does one thing with them, and returns a recent consequence sign.
A Redux reducer characteristic is precisely the identical belief as this “minimize callback” characteristic! It takes a “previous consequence” (the assert
), and the “most modern merchandise” (the action
object), decides a recent assert sign in accordance to those arguments, and returns that recent assert.
If we had been to receive an array of Redux actions, name minimize()
, and tear in a reducer characteristic, we might maybe presumably well get a the identical system:
const actions = [
{ type: ‘counter/increment’ },
{ type: ‘counter/increment’ },
{ type: ‘counter/increment’ }
]
const initialState = { sign: 0 }
const finalResult = actions.minimize(counterReducer, initialState)
console.log(finalResult)
We can pronounce that Redux reducers minimize a online page online of actions (over time) into a single assert. The variation is that with Array.minimize()
it occurs , and with Redux, it occurs over the lifetime of your working app.
Store
The most modern Redux software assert lives in an object called the store .
The shop is created by passing in a reducer, and has a system called getState
that returns the most modern assert sign:
import { configureStore } from ‘@reduxjs/toolkit’
const store = configureStore({ reducer: counterReducer })
console.log(store.getState())
Dispatch
The Redux store has a system called dispatch
. The most productive system to interchange the assert is to name store.dispatch()
and tear in an action object. The shop will bustle its reducer characteristic and establish the recent assert sign inner, and we are able to name getState()
to retrieve the updated sign:
store.dispatch({ variety: ‘counter/increment’ })
console.log(store.getState())
You might maybe presumably maybe presumably judge of dispatching actions as “triggering an match” in the software. One thing took online page online, and we need the shop to know about it. Reducers act fancy match listeners, and when they hear an action they are attracted to, they change the assert in response.
We in total name action creators to dispatch the upright action:
const increment = () => {
return {
variety: ‘counter/increment’
}
}
store.dispatch(increment())
console.log(store.getState())
Selectors
Selectors are capabilities that know pointers on how to extract speak pieces of recordsdata from a store assert sign. As an software grows bigger, this can encourage steer determined of repeating common sense as varied parts of the app opt to learn the identical recordsdata:
const selectCounterValue = assert => assert.sign
const currentValue = selectCounterValue(store.getState())
console.log(currentValue)
Redux Application Knowledge Float
Earlier, we talked about “one-system recordsdata drift”, which describes this sequence of steps to interchange the app:
- Deliver describes the location of the app at a particular point in time
- The UI is rendered in accordance to that assert
- When one thing occurs (just like a person clicking a button), the assert is updated in accordance to what took place
- The UI re-renders in accordance to the recent assert
For Redux namely, we are able to shatter these steps into more part:
- Initial setup:
- A Redux store is created the utilize of a root reducer characteristic
- The shop calls the inspiration reducer as soon as, and saves the return sign as its initial
assert
- When the UI is first rendered, UI substances entry the most modern assert of the Redux store, and utilize that recordsdata to make a decision what to render. They moreover subscribe to any future store updates so that they are able to know if the assert has changed.
- Updates:
- One thing occurs in the app, just like a person clicking a button
- The app code dispatches an action to the Redux store, fancy
dispatch({variety: 'counter/increment'})
- The shop runs the reducer characteristic again with the previous
assert
and the most modernaction
, and saves the return sign as the recentassert
- The shop notifies all parts of the UI which would perhaps presumably maybe presumably be subscribed that the shop has been updated
- Each UI part that needs recordsdata from the shop checks to leer if the parts of the assert they need come by changed.
- Each part that sees its recordsdata has changed forces a re-render with the recent recordsdata, so it might maybe most likely presumably maybe change what’s proven on the cover
Right here’s what that recordsdata drift looks fancy visually:
What You come by Discovered
Redux does come by a preference of most modern phrases and concepts to have in mind. As a reminder, here’s what we excellent covered:
tip
- Redux is a library for managing world software assert
- Redux is in total extinct with the React-Redux library for integrating Redux and React collectively
- Redux Toolkit is the in actual fact useful system to jot down Redux common sense
- Redux uses a “one-system recordsdata drift” app structure
- Deliver describes the location of the app at a level in time, and UI renders in accordance to that assert
- When one thing occurs in the app:
- The UI dispatches an action
- The shop runs the reducers, and the assert is updated in accordance to what took place
- The shop notifies the UI that the assert has changed
- The UI re-renders in accordance to the recent assert
- Redux uses quite loads of forms of code
- Actions are undeniable objects with a
variety
discipline, and record “what took online page online” in the app - Reducers are capabilities that calculate a recent assert sign in accordance to previous assert + an action
- A Redux store runs the inspiration reducer whenever an action is dispatched
- Actions are undeniable objects with a
What’s Subsequent?
We come by considered every of the actual person pieces of a Redux app. Subsequent, continue on to Part 2: Redux App Structure, where we will sign at a plump working instance to leer how the pieces fit collectively.